JavaScript 是前端核心, 掌握这门语言是步入前端高手行列必经之路,噢,别忘了还有TypeScript, 学习它还需要OOP知识, 底层的浏览器原理、HTTP协议也必不可少, 此系列文章记录使用JavaScript和Canvas进行游戏开发, 有游戏才有趣!!!
飞鸟游戏
- 技术要点
- 盒子计算方式box-sizing
- Flex布局
- vh,vw,vmin单位
- 变量定义和访问
- 计算calc
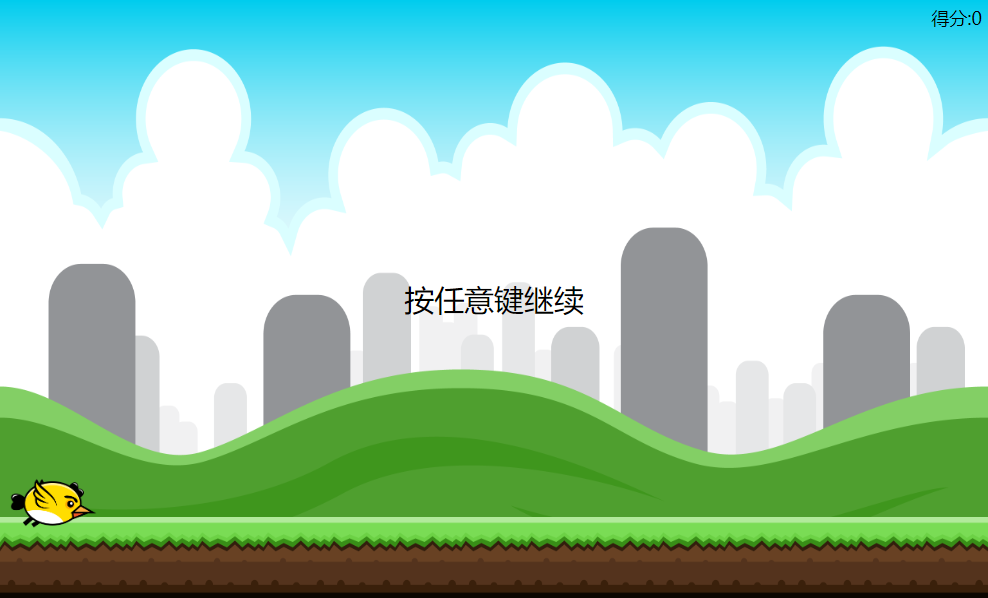
一、游戏主界面设计
- 创建一个HTML5页面
- 盒子的大小的计算方式为box-sizing: border-box
- 用户无法选择 user-select: none
- 所有元素按默认的排列方式排列
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>飞鸟</title> <style> *, *::before, *::after { box-sizing: border-box; user-select: none; } </style> </head> <body> <div class="city" id="city"> <div class="score">得分:<span>0</span></div> <div class="start-control">按任意键继续</div> <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/frame-1.png" class="bird"> </div> </body> </html>
|
二、添加body样式
- 整个body设置为flex布局, 元素居中显示
- 最小高度设置为 100vh
- 此时的页面内容会超出屏幕的显示区域,也就是说有纵向和横向的滚动条
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>飞鸟</title> <style> *, *::before, *::after { box-sizing: border-box; user-select: none; } body { margin: 0; display: flex; justify-content: center; align-items: center; min-height: 100vh; } </style> </head> <body> <div class="city" id="city"> <div class="score">得分:<span>0</span></div> <div class="start-control">按任意键继续</div> <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/frame-1.png" class="bird"> </div> </body> </html>
|
二、添加city样式
- 在div.city中固定宽度和高度,同时让超出显示区域的内容隐藏
- position设定为relative
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>飞鸟</title> <style> *, *::before, *::after { box-sizing: border-box; user-select: none; } body { margin: 0; display: flex; justify-content: center; align-items: center; min-height: 100vh; } .city { overflow: hidden; position: relative; width: 100vw; height: 100vh; } </style> </head> <body> <div class="city" id="city"> <div class="score">得分:<span>0</span></div> <div class="start-control">按任意键继续</div> <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/frame-1.png" class="bird"> </div> </body> </html>
|
三、div.score样式设计
- 得分设计在右上角的位置,需要定义position为absolute以及top和right属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>飞鸟</title> <style> *, *::before, *::after { box-sizing: border-box; user-select: none; } body { margin: 0; display: flex; justify-content: center; align-items: center; min-height: 100vh; } .city { overflow: hidden; position: relative; width: 100vw; height: 100vh; } .score { position: absolute; font-size: 3vmin; right: 1vmin; top: 1vmin; } </style> </head> <body> <div class="city" id="city"> <div class="score">得分:<span>0</span></div> <div class="start-control">按任意键继续</div> <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/frame-1.png" class="bird"> </div> </body> </html>
|
四、定义开始游戏的提示文本样式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>飞鸟</title> <style> .start-control { position: absolute; font-size: 5vmin; top: 50%; left: 50%; transform: translate(-50%, -50%); } </style> </head> <body> <div class="city" id="city"> <div class="score">得分:<span>0</span></div> <div class="start-control">按任意键继续</div> <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/frame-1.png" class="bird"> </div> </body> </html>
|
五、设置背景图片样式
- 使用css3中定义的样式变量 –left
- 使用calc函数来计算 left的值, left的值后续需要动态变化, 每次变化的值是 –left设定的值的 百分之一, 这个值也可改成任意百分比
- 宽度充满屏幕可见区域
- 高度设置为100vh
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>飞鸟</title> <style> .bg_ground { --left: 0; position: absolute; width: 100%; height: 100vh; bottom: 0; left: calc(var(--left) * 1%); } </style> </head> <body> <div class="city" id="city"> <div class="score">得分:<span>0</span></div> <div class="start-control">按任意键继续</div> <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/frame-1.png" class="bird"> </div> </body> </html>
|
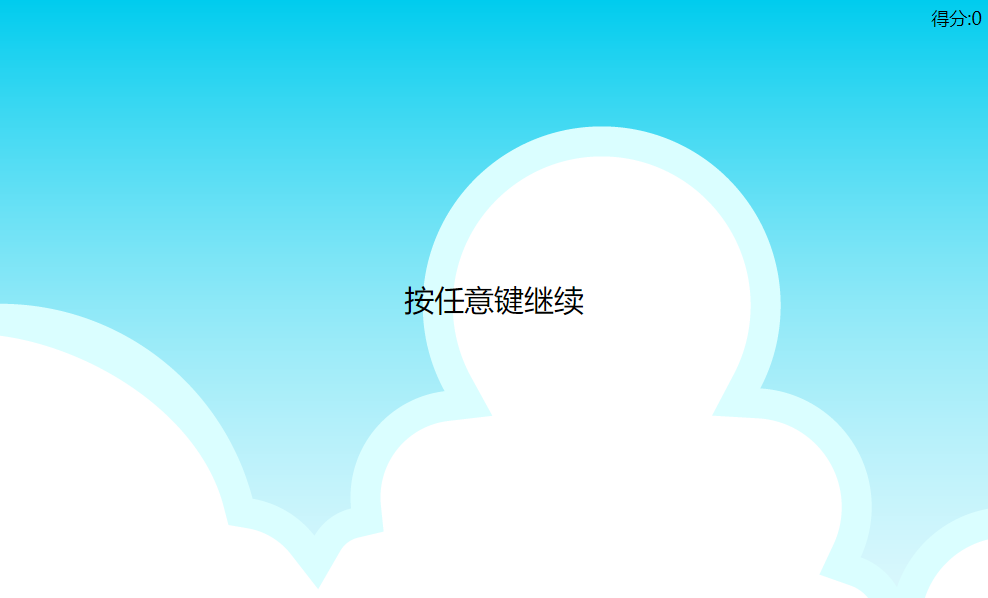
六、主角飞鸟样式设计
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>飞鸟</title> <style> .bird { --bottom: 10; position: absolute; left: 1%; height: 10%; bottom: calc(var(--bottom) * 1%); } </style> </head> <body> <div class="city" id="city"> <div class="score">得分:<span>0</span></div> <div class="start-control">按任意键继续</div> <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/full-background.png" class="bg_ground" > <img src="imgs/frame-1.png" class="bird"> </div> </body> </html>
|
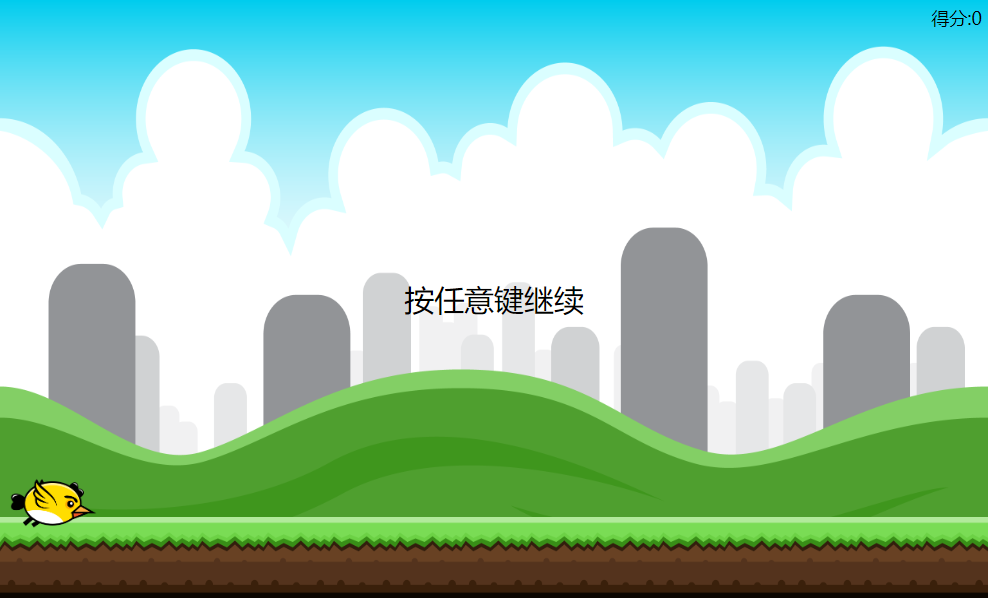
如果希望角色能够动起来,需要添加JS脚本,先看下图, 具体怎样做下篇见分晓
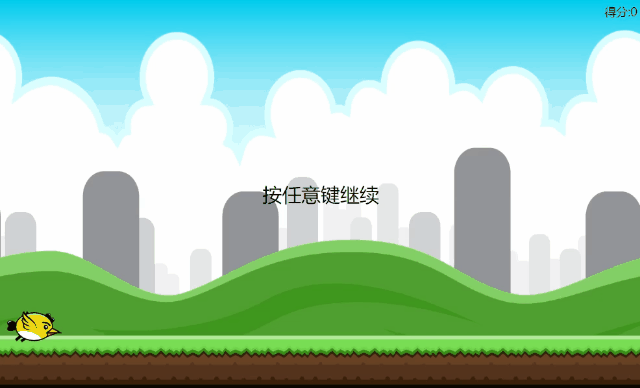